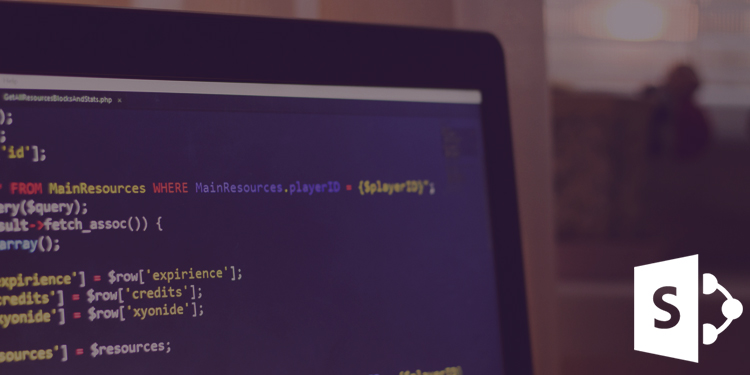
In the real world, the HR department is responsible in any organization to appoint new employee. Once he/she appoints any employee then he/she requires to contact Network Administrator and SharePoint Administrator. A Network Administrator creates this user in the AD, assign him into OU (if require) and AD group, and a SharePoint Administrator assigns this user into appropriate SharePoint group to provide him access on the organization SharePoint site. Thus, a chronological sequence is required to follow and it is time-consuming.
If HR department is having appropriate permission to access the organization SharePoint site then they can perform the tasks on behalf of Network and SharePoint Administrators through SharePoint itself via a web-part, an application page or an event receiver. Thus, the HR department can get rid of the above chronological sequence.
Before providing access to a new user in a SharePoint site, an organized user is required to perform certain steps as mentioned below:
- Create a user in Active Directory
- Add a user in Organizational Unit (if require)
- Add a user in Active Directory group
- Add a user in a SharePoint group
Ideally, the Active Directory tasks (steps 1 to 3) are performed by a user who is having permission on Active Directory Domain Services. Step 4 can be performed by a user who is having access to manage users in SharePoint groups of a SharePoint site.
Let’s see how one can provide this functionality in SharePoint site.
Note: The yellow highlighted points in below code are configurable as per the user requirement. This information must be kept somewhere where one can easily manage them. E.g. web.config, app.config, SharePoint list etc.
Code Example
Let’s create a console application in C# to create users in the AD and assign them into SharePoint Group. The developer can also add a piece of code in SharePoint web-part, an application page or an event receiver with required modification.
First, get all the required user information from an end user and use them to create a user.
Try { // fetch the user details from input Console.Write("Enter First name: "); var firstName = Console.ReadLine(); Console.Write("Enter Last name: "); var lastName = Console.ReadLine(); Console.Write("Enter Login name: "); var loginName = Console.ReadLine(); Console.Write("Enter Employee ID: "); var employeeID = Console.ReadLine(); Console.Write("Enter Email: "); var email = Console.ReadLine(); Console.Write("Enter Phone number: "); var phoneNumber = Console.ReadLine(); Console.Write("Enter Address: "); var address = Console.ReadLine(); AddUserInSharePoint(firstName, lastName, loginName, employeeID, email, phoneNumber, address); } catch (Exception ex) { Console.WriteLine("Error: " + ex.Message); } Console.ReadLine(); |
Add User in SharePoint
public static void AddUserInSharePoint(string firstName, string lastName, string userLogonName, string employeeID, string emailAddress, string telephone, string address) { try { bool status = CreateUser(firstName, lastName, loginName, employeeID, email, phoneNumber, address); if (status) { PrincipalContext AD = new PrincipalContext(ContextType.Domain, "your domain", "User Name", "Password"); UserPrincipal u = new UserPrincipal(AD); // search for user PrincipalSearcher search = new PrincipalSearcher(u); UserPrincipal result = (UserPrincipal)search.FindOne(); search.Dispose(); //Enter user to SharePoint group if (result != null) { AddUserToDirectoryGroup(result.UserPrincipalName, "AD Group Name", "Your Domain", "DC=DC,DC=DC"); AddUserToSharePointGroup(result.SamAccountName, "SP Group Name"); // SamAccountName = User's AD login name } } } catch (DirectoryServicesCOMException ex) { Console.WriteLine("Error: " + ex.Message); } } |
Create User
private static bool CreateUser(string firstName, string lastName, string userLogonName, string employeeID, string emailAddress, string telephone, string address) { // Creating the PrincipalContext PrincipalContext principalContext = null; try { principalContext = new PrincipalContext(ContextType.Domain, "your domain", "DC=DC,DC=DC"); } catch (Exception ex) { Console.WriteLine("Error: " + ex.Message); throw ex; } // Check if user object already exists in the store UserPrincipal usr = UserPrincipal.FindByIdentity(principalContext, userLogonName); if (usr != null) { return false; } // Create the new UserPrincipal object UserPrincipal userPrincipal = new UserPrincipal(principalContext); if (lastName != null && lastName.Length > 0) { userPrincipal.Surname = lastName; } if (firstName != null && firstName.Length > 0) { userPrincipal.GivenName = firstName; } if (employeeID != null && employeeID.Length > 0) { userPrincipal.EmployeeId = employeeID; } if (emailAddress != null && emailAddress.Length > 0) { userPrincipal.EmailAddress = emailAddress; } if (telephone != null && telephone.Length > 0) { userPrincipal.VoiceTelephoneNumber = telephone; } if (userLogonName != null && userLogonName.Length > 0) { userPrincipal.SamAccountName = userLogonName; } string pwdOfNewlyCreatedUser = "abcde@@12345!~"; //Random or auto-generated password can be assigned. userPrincipal.SetPassword(pwdOfNewlyCreatedUser); userPrincipal.Enabled = true; userPrincipal.ExpirePasswordNow(); try { userPrincipal.Save(); } catch (Exception ex) { Console.WriteLine("Error: " + ex.Message); return false; } if (userPrincipal.GetUnderlyingObjectType() == typeof(DirectoryEntry)) { DirectoryEntry entry = (DirectoryEntry)userPrincipal.GetUnderlyingObject(); if (address != null && address.Length > 0) { entry.Properties["streetAddress"].Value = address; } try { entry.CommitChanges(); } catch (Exception ex) { Console.WriteLine("Error: " + ex.Message); return false; } } return true; } |
Add User to Directory Group
public static void AddUserToDirectoryGroup(string userId, string groupName, string domain, string domainController) { try { using (PrincipalContext pc = new PrincipalContext(ContextType.Domain, domain, domainController)) { GroupPrincipal group = GroupPrincipal.FindByIdentity(pc, groupName); group.Members.Add(pc, IdentityType.UserPrincipalName, userId); group.Save(); } } catch (DirectoryServicesCOMException ex) { Console.WriteLine("Error: " + ex.Message); } } |
Add User to SharePoint Group
private static void AddUserToSharePointGroup(string userLoginName, string userGroupName) { //Executes this method with Full Control rights even if the user does not otherwise have Full Control SPSecurity.RunWithElevatedPrivileges(delegate { using (SPSite spSite = new SPSite("Your site url")) { using (SPWeb spWeb = spSite.OpenWeb()) { try { //Allow updating of some sharepoint lists, (here spUsers, spGroups etc...) spWeb.AllowUnsafeUpdates = true; SPUser spUser = spWeb.EnsureUser(userLoginName); if (spUser != null) { SPGroup spGroup = spWeb.Groups[userGroupName]; if (spGroup != null) { spGroup.AddUser(spUser); } } } catch (Exception ex) { Console.WriteLine("Error: " + ex.Message); } finally { spWeb.AllowUnsafeUpdates = false; } } } }); } |
Output
On successful execution of the AddUserInSharePoint method, the user will be added to the Active directory. You can verify the same by logging into the Active Directory. Refer the below screenshots for same.
On completion of AddUserToSharePointGroup method, the user will be added to a SharePoint group as shown in the screenshot below.
Conclusion
A new user can be created and assigned into the respective AD and SharePoint groups by a user who is having appropriate permission on a SharePoint site. The various information related to the user which is provided at a creation time can be viewed in SharePoint if the User Profile Synchronization Service is configured. Various operations can be performed in AD and SharePoint related to a user management.
Comments
Leave a message...